Notifications
- Introduction
- Generating Notifications
- Sending Notifications
- Mail Notifications
- Markdown Mail Notifications
- Database Notifications
- Broadcast Notifications
- SMS Notifications
- Slack Notifications
- Localizing Notifications
- Notification Events
- Custom Channels
Introduction
In addition to support for sending email, Laravel provides support for sending notifications across a variety of delivery channels, including email, SMS (via Vonage, formerly known as Nexmo), and Slack. In addition, a variety of community built notification channels have been created to send notifications over dozens of different channels! Notifications may also be stored in a database so they may be displayed in your web interface.
Typically, notifications should be short, informational messages that notify users of something that occurred in your application. For example, if you are writing a billing application, you might send an "Invoice Paid" notification to your users via the email and SMS channels.
Generating Notifications
In Laravel, each notification is represented by a single class that is typically stored in the app/Notifications
directory. Don't worry if you don't see this directory in your application - it will be created for you when you run the make:notification
Artisan command:
1php artisan make:notification InvoicePaid
This command will place a fresh notification class in your app/Notifications
directory. Each notification class contains a via
method and a variable number of message building methods, such as toMail
or toDatabase
, that convert the notification to a message tailored for that particular channel.
Sending Notifications
Using The Notifiable Trait
Notifications may be sent in two ways: using the notify
method of the Notifiable
trait or using the Notification
facade. The Notifiable
trait is included on your application's App\Models\User
model by default:
1<?php 2 3namespace App\Models; 4 5use Illuminate\Foundation\Auth\User as Authenticatable; 6use Illuminate\Notifications\Notifiable; 7 8class User extends Authenticatable 9{10 use Notifiable;11}
The notify
method that is provided by this trait expects to receive a notification instance:
1use App\Notifications\InvoicePaid;2 3$user->notify(new InvoicePaid($invoice));
Remember, you may use the Notifiable
trait on any of your models. You are not limited to only including it on your User
model.
Using The Notification Facade
Alternatively, you may send notifications via the Notification
facade. This approach is useful when you need to send a notification to multiple notifiable entities such as a collection of users. To send notifications using the facade, pass all of the notifiable entities and the notification instance to the send
method:
1use Illuminate\Support\Facades\Notification;2 3Notification::send($users, new InvoicePaid($invoice));
You can also send notifications immediately using the sendNow
method. This method will send the notification immediately even if the notification implements the ShouldQueue
interface:
1Notification::sendNow($developers, new DeploymentCompleted($deployment));
Specifying Delivery Channels
Every notification class has a via
method that determines on which channels the notification will be delivered. Notifications may be sent on the mail
, database
, broadcast
, vonage
, and slack
channels.
If you would like to use other delivery channels such as Telegram or Pusher, check out the community driven Laravel Notification Channels website.
The via
method receives a $notifiable
instance, which will be an instance of the class to which the notification is being sent. You may use $notifiable
to determine which channels the notification should be delivered on:
1/** 2 * Get the notification's delivery channels. 3 * 4 * @param mixed $notifiable 5 * @return array 6 */ 7public function via($notifiable) 8{ 9 return $notifiable->prefers_sms ? ['vonage'] : ['mail', 'database'];10}
Queueing Notifications
Before queueing notifications you should configure your queue and start a worker.
Sending notifications can take time, especially if the channel needs to make an external API call to deliver the notification. To speed up your application's response time, let your notification be queued by adding the ShouldQueue
interface and Queueable
trait to your class. The interface and trait are already imported for all notifications generated using the make:notification
command, so you may immediately add them to your notification class:
1<?php 2 3namespace App\Notifications; 4 5use Illuminate\Bus\Queueable; 6use Illuminate\Contracts\Queue\ShouldQueue; 7use Illuminate\Notifications\Notification; 8 9class InvoicePaid extends Notification implements ShouldQueue10{11 use Queueable;12 13 // ...14}
Once the ShouldQueue
interface has been added to your notification, you may send the notification like normal. Laravel will detect the ShouldQueue
interface on the class and automatically queue the delivery of the notification:
1$user->notify(new InvoicePaid($invoice));
When queueing notifications, a queued job will be created for each recipient and channel combination. For example, six jobs will be dispatched to the queue if your notification has three recipients and two channels.
Delaying Notifications
If you would like to delay the delivery of the notification, you may chain the delay
method onto your notification instantiation:
1$delay = now()->addMinutes(10);2 3$user->notify((new InvoicePaid($invoice))->delay($delay));
Delaying Notifications Per Channel
You may pass an array to the delay
method to specify the delay amount for specific channels:
1$user->notify((new InvoicePaid($invoice))->delay([2 'mail' => now()->addMinutes(5),3 'sms' => now()->addMinutes(10),4]));
Alternatively, you may define a withDelay
method on the notification class itself. The withDelay
method should return an array of channel names and delay values:
1/** 2 * Determine the notification's delivery delay. 3 * 4 * @param mixed $notifiable 5 * @return array 6 */ 7public function withDelay($notifiable) 8{ 9 return [10 'mail' => now()->addMinutes(5),11 'sms' => now()->addMinutes(10),12 ];13}
Customizing The Notification Queue Connection
By default, queued notifications will be queued using your application's default queue connection. If you would like to specify a different connection that should be used for a particular notification, you may define a $connection
property on the notification class:
1/**2 * The name of the queue connection to use when queueing the notification.3 *4 * @var string5 */6public $connection = 'redis';
Or, if you would like to specify a specific queue connection that should be used for each notification channel supported by the notification, you may define a viaConnections
method on your notification. This method should return an array of channel name / queue connection name pairs:
1/** 2 * Determine which connections should be used for each notification channel. 3 * 4 * @return array 5 */ 6public function viaConnections() 7{ 8 return [ 9 'mail' => 'redis',10 'database' => 'sync',11 ];12}
Customizing Notification Channel Queues
If you would like to specify a specific queue that should be used for each notification channel supported by the notification, you may define a viaQueues
method on your notification. This method should return an array of channel name / queue name pairs:
1/** 2 * Determine which queues should be used for each notification channel. 3 * 4 * @return array 5 */ 6public function viaQueues() 7{ 8 return [ 9 'mail' => 'mail-queue',10 'slack' => 'slack-queue',11 ];12}
Queued Notifications & Database Transactions
When queued notifications are dispatched within database transactions, they may be processed by the queue before the database transaction has committed. When this happens, any updates you have made to models or database records during the database transaction may not yet be reflected in the database. In addition, any models or database records created within the transaction may not exist in the database. If your notification depends on these models, unexpected errors can occur when the job that sends the queued notification is processed.
If your queue connection's after_commit
configuration option is set to false
, you may still indicate that a particular queued notification should be dispatched after all open database transactions have been committed by calling the afterCommit
method when sending the notification:
1use App\Notifications\InvoicePaid;2 3$user->notify((new InvoicePaid($invoice))->afterCommit());
Alternatively, you may call the afterCommit
method from your notification's constructor:
1<?php 2 3namespace App\Notifications; 4 5use Illuminate\Bus\Queueable; 6use Illuminate\Contracts\Queue\ShouldQueue; 7use Illuminate\Notifications\Notification; 8 9class InvoicePaid extends Notification implements ShouldQueue10{11 use Queueable;12 13 /**14 * Create a new notification instance.15 *16 * @return void17 */18 public function __construct()19 {20 $this->afterCommit();21 }22}
To learn more about working around these issues, please review the documentation regarding queued jobs and database transactions.
Determining If A Queued Notification Should Be Sent
After a queued notification has been dispatched for the queue for background processing, it will typically be accepted by a queue worker and sent to its intended recipient.
However, if you would like to make the final determination on whether the queued notification should be sent after it is being processed by a queue worker, you may define a shouldSend
method on the notification class. If this method returns false
, the notification will not be sent:
1/** 2 * Determine if the notification should be sent. 3 * 4 * @param mixed $notifiable 5 * @param string $channel 6 * @return bool 7 */ 8public function shouldSend($notifiable, $channel) 9{10 return $this->invoice->isPaid();11}
On-Demand Notifications
Sometimes you may need to send a notification to someone who is not stored as a "user" of your application. Using the Notification
facade's route
method, you may specify ad-hoc notification routing information before sending the notification:
1use Illuminate\Broadcasting\Channel;2use Illuminate\Support\Facades\Notification;3 4Notification::route('mail', 'taylor@example.com')5 ->route('vonage', '5555555555')6 ->route('slack', 'https://hooks.slack.com/services/...')7 ->route('broadcast', [new Channel('channel-name')])8 ->notify(new InvoicePaid($invoice));
If you would like to provide the recipient's name when sending an on-demand notification to the mail
route, you may provide an array that contains the email address as the key and the name as the value of the first element in the array:
1Notification::route('mail', [2 'barrett@example.com' => 'Barrett Blair',3])->notify(new InvoicePaid($invoice));
Mail Notifications
Formatting Mail Messages
If a notification supports being sent as an email, you should define a toMail
method on the notification class. This method will receive a $notifiable
entity and should return an Illuminate\Notifications\Messages\MailMessage
instance.
The MailMessage
class contains a few simple methods to help you build transactional email messages. Mail messages may contain lines of text as well as a "call to action". Let's take a look at an example toMail
method:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 $url = url('/invoice/'.$this->invoice->id);10 11 return (new MailMessage)12 ->greeting('Hello!')13 ->line('One of your invoices has been paid!')14 ->lineIf($this->amount > 0, "Amount paid: {$this->amount}")15 ->action('View Invoice', $url)16 ->line('Thank you for using our application!');17}
Note we are using $this->invoice->id
in our toMail
method. You may pass any data your notification needs to generate its message into the notification's constructor.
In this example, we register a greeting, a line of text, a call to action, and then another line of text. These methods provided by the MailMessage
object make it simple and fast to format small transactional emails. The mail channel will then translate the message components into a beautiful, responsive HTML email template with a plain-text counterpart. Here is an example of an email generated by the mail
channel:
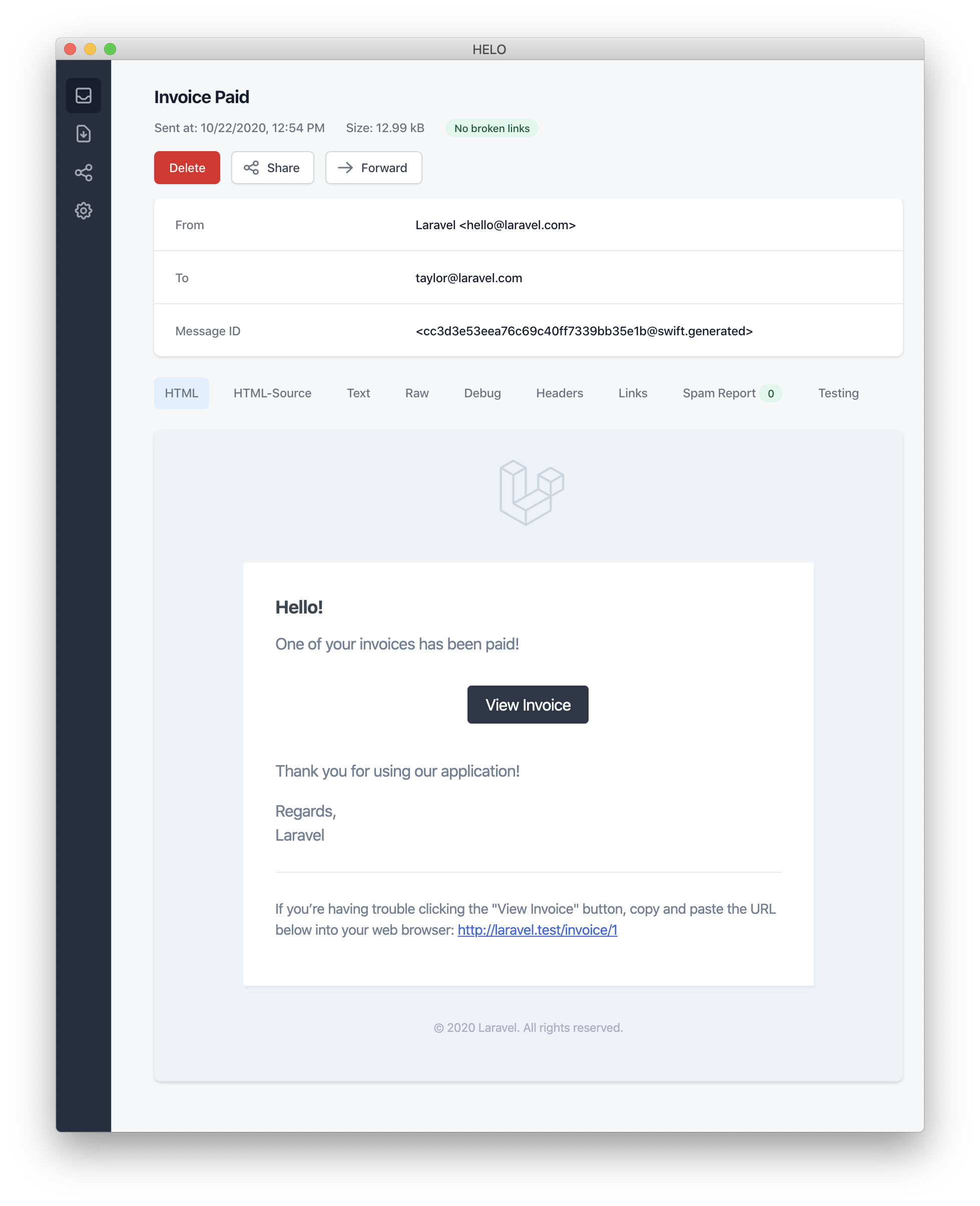
When sending mail notifications, be sure to set the name
configuration option in your config/app.php
configuration file. This value will be used in the header and footer of your mail notification messages.
Error Messages
Some notifications inform users of errors, such as a failed invoice payment. You may indicate that a mail message is regarding an error by calling the error
method when building your message. When using the error
method on a mail message, the call to action button will be red instead of black:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->error()11 ->subject('Invoice Payment Failed')12 ->line('...');13}
Other Mail Notification Formatting Options
Instead of defining the "lines" of text in the notification class, you may use the view
method to specify a custom template that should be used to render the notification email:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)->view(10 'emails.name', ['invoice' => $this->invoice]11 );12}
You may specify a plain-text view for the mail message by passing the view name as the second element of an array that is given to the view
method:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)->view(10 ['emails.name.html', 'emails.name.plain'],11 ['invoice' => $this->invoice]12 );13}
Customizing The Sender
By default, the email's sender / from address is defined in the config/mail.php
configuration file. However, you may specify the from address for a specific notification using the from
method:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->from('barrett@example.com', 'Barrett Blair')11 ->line('...');12}
Customizing The Recipient
When sending notifications via the mail
channel, the notification system will automatically look for an email
property on your notifiable entity. You may customize which email address is used to deliver the notification by defining a routeNotificationForMail
method on the notifiable entity:
1<?php 2 3namespace App\Models; 4 5use Illuminate\Foundation\Auth\User as Authenticatable; 6use Illuminate\Notifications\Notifiable; 7 8class User extends Authenticatable 9{10 use Notifiable;11 12 /**13 * Route notifications for the mail channel.14 *15 * @param \Illuminate\Notifications\Notification $notification16 * @return array|string17 */18 public function routeNotificationForMail($notification)19 {20 // Return email address only...21 return $this->email_address;22 23 // Return email address and name...24 return [$this->email_address => $this->name];25 }26}
Customizing The Subject
By default, the email's subject is the class name of the notification formatted to "Title Case". So, if your notification class is named InvoicePaid
, the email's subject will be Invoice Paid
. If you would like to specify a different subject for the message, you may call the subject
method when building your message:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->subject('Notification Subject')11 ->line('...');12}
Customizing The Mailer
By default, the email notification will be sent using the default mailer defined in the config/mail.php
configuration file. However, you may specify a different mailer at runtime by calling the mailer
method when building your message:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->mailer('postmark')11 ->line('...');12}
Customizing The Templates
You can modify the HTML and plain-text template used by mail notifications by publishing the notification package's resources. After running this command, the mail notification templates will be located in the resources/views/vendor/notifications
directory:
1php artisan vendor:publish --tag=laravel-notifications
Attachments
To add attachments to an email notification, use the attach
method while building your message. The attach
method accepts the absolute path to the file as its first argument:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->greeting('Hello!')11 ->attach('/path/to/file');12}
The attach
method offered by notification mail messages also accepts attachable objects. Please consult the comprehensive attachable object documentation to learn more.
When attaching files to a message, you may also specify the display name and / or MIME type by passing an array
as the second argument to the attach
method:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->greeting('Hello!')11 ->attach('/path/to/file', [12 'as' => 'name.pdf',13 'mime' => 'application/pdf',14 ]);15}
Unlike attaching files in mailable objects, you may not attach a file directly from a storage disk using attachFromStorage
. You should rather use the attach
method with an absolute path to the file on the storage disk. Alternatively, you could return a mailable from the toMail
method:
1use App\Mail\InvoicePaid as InvoicePaidMailable; 2 3/** 4 * Get the mail representation of the notification. 5 * 6 * @param mixed $notifiable 7 * @return Mailable 8 */ 9public function toMail($notifiable)10{11 return (new InvoicePaidMailable($this->invoice))12 ->to($notifiable->email)13 ->attachFromStorage('/path/to/file');14}
When necessary, multiple files may be attached to a message using the attachMany
method:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->greeting('Hello!')11 ->attachMany([12 '/path/to/forge.svg',13 '/path/to/vapor.svg' => [14 'as' => 'Logo.svg',15 'mime' => 'image/svg+xml',16 ],17 ]);18}
Raw Data Attachments
The attachData
method may be used to attach a raw string of bytes as an attachment. When calling the attachData
method, you should provide the filename that should be assigned to the attachment:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->greeting('Hello!')11 ->attachData($this->pdf, 'name.pdf', [12 'mime' => 'application/pdf',13 ]);14}
Adding Tags & Metadata
Some third-party email providers such as Mailgun and Postmark support message "tags" and "metadata", which may be used to group and track emails sent by your application. You may add tags and metadata to an email message via the tag
and metadata
methods:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->greeting('Comment Upvoted!')11 ->tag('upvote')12 ->metadata('comment_id', $this->comment->id);13}
If your application is using the Mailgun driver, you may consult Mailgun's documentation for more information on tags and metadata. Likewise, the Postmark documentation may also be consulted for more information on their support for tags and metadata.
If your application is using Amazon SES to send emails, you should use the metadata
method to attach SES "tags" to the message.
Customizing The Symfony Message
The withSymfonyMessage
method of the MailMessage
class allows you to register a closure which will be invoked with the Symfony Message instance before sending the message. This gives you an opportunity to deeply customize the message before it is delivered:
1use Symfony\Component\Mime\Email; 2 3/** 4 * Get the mail representation of the notification. 5 * 6 * @param mixed $notifiable 7 * @return \Illuminate\Notifications\Messages\MailMessage 8 */ 9public function toMail($notifiable)10{11 return (new MailMessage)12 ->withSymfonyMessage(function (Email $message) {13 $message->getHeaders()->addTextHeader(14 'Custom-Header', 'Header Value'15 );16 });17}
Using Mailables
If needed, you may return a full mailable object from your notification's toMail
method. When returning a Mailable
instead of a MailMessage
, you will need to specify the message recipient using the mailable object's to
method:
1use App\Mail\InvoicePaid as InvoicePaidMailable; 2 3/** 4 * Get the mail representation of the notification. 5 * 6 * @param mixed $notifiable 7 * @return Mailable 8 */ 9public function toMail($notifiable)10{11 return (new InvoicePaidMailable($this->invoice))12 ->to($notifiable->email);13}
Mailables & On-Demand Notifications
If you are sending an on-demand notification, the $notifiable
instance given to the toMail
method will be an instance of Illuminate\Notifications\AnonymousNotifiable
, which offers a routeNotificationFor
method that may be used to retrieve the email address the on-demand notification should be sent to:
1use App\Mail\InvoicePaid as InvoicePaidMailable; 2use Illuminate\Notifications\AnonymousNotifiable; 3 4/** 5 * Get the mail representation of the notification. 6 * 7 * @param mixed $notifiable 8 * @return Mailable 9 */10public function toMail($notifiable)11{12 $address = $notifiable instanceof AnonymousNotifiable13 ? $notifiable->routeNotificationFor('mail')14 : $notifiable->email;15 16 return (new InvoicePaidMailable($this->invoice))17 ->to($address);18}
Previewing Mail Notifications
When designing a mail notification template, it is convenient to quickly preview the rendered mail message in your browser like a typical Blade template. For this reason, Laravel allows you to return any mail message generated by a mail notification directly from a route closure or controller. When a MailMessage
is returned, it will be rendered and displayed in the browser, allowing you to quickly preview its design without needing to send it to an actual email address:
1use App\Models\Invoice;2use App\Notifications\InvoicePaid;3 4Route::get('/notification', function () {5 $invoice = Invoice::find(1);6 7 return (new InvoicePaid($invoice))8 ->toMail($invoice->user);9});
Markdown Mail Notifications
Markdown mail notifications allow you to take advantage of the pre-built templates of mail notifications, while giving you more freedom to write longer, customized messages. Since the messages are written in Markdown, Laravel is able to render beautiful, responsive HTML templates for the messages while also automatically generating a plain-text counterpart.
Generating The Message
To generate a notification with a corresponding Markdown template, you may use the --markdown
option of the make:notification
Artisan command:
1php artisan make:notification InvoicePaid --markdown=mail.invoice.paid
Like all other mail notifications, notifications that use Markdown templates should define a toMail
method on their notification class. However, instead of using the line
and action
methods to construct the notification, use the markdown
method to specify the name of the Markdown template that should be used. An array of data you wish to make available to the template may be passed as the method's second argument:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 $url = url('/invoice/'.$this->invoice->id);10 11 return (new MailMessage)12 ->subject('Invoice Paid')13 ->markdown('mail.invoice.paid', ['url' => $url]);14}
Writing The Message
Markdown mail notifications use a combination of Blade components and Markdown syntax which allow you to easily construct notifications while leveraging Laravel's pre-crafted notification components:
1<x-mail::message> 2# Invoice Paid 3 4Your invoice has been paid! 5 6<x-mail::button :url="$url"> 7View Invoice 8</x-mail::button> 9 10Thanks,<br>11{{ config('app.name') }}12</x-mail::message>
Button Component
The button component renders a centered button link. The component accepts two arguments, a url
and an optional color
. Supported colors are primary
, green
, and red
. You may add as many button components to a notification as you wish:
1<x-mail::button :url="$url" color="green">2View Invoice3</x-mail::button>
Panel Component
The panel component renders the given block of text in a panel that has a slightly different background color than the rest of the notification. This allows you to draw attention to a given block of text:
1<x-mail::panel>2This is the panel content.3</x-mail::panel>
Table Component
The table component allows you to transform a Markdown table into an HTML table. The component accepts the Markdown table as its content. Table column alignment is supported using the default Markdown table alignment syntax:
1<x-mail::table>2| Laravel | Table | Example |3| ------------- |:-------------:| --------:|4| Col 2 is | Centered | $10 |5| Col 3 is | Right-Aligned | $20 |6</x-mail::table>
Customizing The Components
You may export all of the Markdown notification components to your own application for customization. To export the components, use the vendor:publish
Artisan command to publish the laravel-mail
asset tag:
1php artisan vendor:publish --tag=laravel-mail
This command will publish the Markdown mail components to the resources/views/vendor/mail
directory. The mail
directory will contain an html
and a text
directory, each containing their respective representations of every available component. You are free to customize these components however you like.
Customizing The CSS
After exporting the components, the resources/views/vendor/mail/html/themes
directory will contain a default.css
file. You may customize the CSS in this file and your styles will automatically be in-lined within the HTML representations of your Markdown notifications.
If you would like to build an entirely new theme for Laravel's Markdown components, you may place a CSS file within the html/themes
directory. After naming and saving your CSS file, update the theme
option of the mail
configuration file to match the name of your new theme.
To customize the theme for an individual notification, you may call the theme
method while building the notification's mail message. The theme
method accepts the name of the theme that should be used when sending the notification:
1/** 2 * Get the mail representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\MailMessage 6 */ 7public function toMail($notifiable) 8{ 9 return (new MailMessage)10 ->theme('invoice')11 ->subject('Invoice Paid')12 ->markdown('mail.invoice.paid', ['url' => $url]);13}
Database Notifications
Prerequisites
The database
notification channel stores the notification information in a database table. This table will contain information such as the notification type as well as a JSON data structure that describes the notification.
You can query the table to display the notifications in your application's user interface. But, before you can do that, you will need to create a database table to hold your notifications. You may use the notifications:table
command to generate a migration with the proper table schema:
1php artisan notifications:table2 3php artisan migrate
Formatting Database Notifications
If a notification supports being stored in a database table, you should define a toDatabase
or toArray
method on the notification class. This method will receive a $notifiable
entity and should return a plain PHP array. The returned array will be encoded as JSON and stored in the data
column of your notifications
table. Let's take a look at an example toArray
method:
1/** 2 * Get the array representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return array 6 */ 7public function toArray($notifiable) 8{ 9 return [10 'invoice_id' => $this->invoice->id,11 'amount' => $this->invoice->amount,12 ];13}
toDatabase
Vs. toArray
The toArray
method is also used by the broadcast
channel to determine which data to broadcast to your JavaScript powered frontend. If you would like to have two different array representations for the database
and broadcast
channels, you should define a toDatabase
method instead of a toArray
method.
Accessing The Notifications
Once notifications are stored in the database, you need a convenient way to access them from your notifiable entities. The Illuminate\Notifications\Notifiable
trait, which is included on Laravel's default App\Models\User
model, includes a notifications
Eloquent relationship that returns the notifications for the entity. To fetch notifications, you may access this method like any other Eloquent relationship. By default, notifications will be sorted by the created_at
timestamp with the most recent notifications at the beginning of the collection:
1$user = App\Models\User::find(1);2 3foreach ($user->notifications as $notification) {4 echo $notification->type;5}
If you want to retrieve only the "unread" notifications, you may use the unreadNotifications
relationship. Again, these notifications will be sorted by the created_at
timestamp with the most recent notifications at the beginning of the collection:
1$user = App\Models\User::find(1);2 3foreach ($user->unreadNotifications as $notification) {4 echo $notification->type;5}
To access your notifications from your JavaScript client, you should define a notification controller for your application which returns the notifications for a notifiable entity, such as the current user. You may then make an HTTP request to that controller's URL from your JavaScript client.
Marking Notifications As Read
Typically, you will want to mark a notification as "read" when a user views it. The Illuminate\Notifications\Notifiable
trait provides a markAsRead
method, which updates the read_at
column on the notification's database record:
1$user = App\Models\User::find(1);2 3foreach ($user->unreadNotifications as $notification) {4 $notification->markAsRead();5}
However, instead of looping through each notification, you may use the markAsRead
method directly on a collection of notifications:
1$user->unreadNotifications->markAsRead();
You may also use a mass-update query to mark all of the notifications as read without retrieving them from the database:
1$user = App\Models\User::find(1);2 3$user->unreadNotifications()->update(['read_at' => now()]);
You may delete
the notifications to remove them from the table entirely:
1$user->notifications()->delete();
Broadcast Notifications
Prerequisites
Before broadcasting notifications, you should configure and be familiar with Laravel's event broadcasting services. Event broadcasting provides a way to react to server-side Laravel events from your JavaScript powered frontend.
Formatting Broadcast Notifications
The broadcast
channel broadcasts notifications using Laravel's event broadcasting services, allowing your JavaScript powered frontend to catch notifications in realtime. If a notification supports broadcasting, you can define a toBroadcast
method on the notification class. This method will receive a $notifiable
entity and should return a BroadcastMessage
instance. If the toBroadcast
method does not exist, the toArray
method will be used to gather the data that should be broadcast. The returned data will be encoded as JSON and broadcast to your JavaScript powered frontend. Let's take a look at an example toBroadcast
method:
1use Illuminate\Notifications\Messages\BroadcastMessage; 2 3/** 4 * Get the broadcastable representation of the notification. 5 * 6 * @param mixed $notifiable 7 * @return BroadcastMessage 8 */ 9public function toBroadcast($notifiable)10{11 return new BroadcastMessage([12 'invoice_id' => $this->invoice->id,13 'amount' => $this->invoice->amount,14 ]);15}
Broadcast Queue Configuration
All broadcast notifications are queued for broadcasting. If you would like to configure the queue connection or queue name that is used to queue the broadcast operation, you may use the onConnection
and onQueue
methods of the BroadcastMessage
:
1return (new BroadcastMessage($data))2 ->onConnection('sqs')3 ->onQueue('broadcasts');
Customizing The Notification Type
In addition to the data you specify, all broadcast notifications also have a type
field containing the full class name of the notification. If you would like to customize the notification type
, you may define a broadcastType
method on the notification class:
1use Illuminate\Notifications\Messages\BroadcastMessage; 2 3/** 4 * Get the type of the notification being broadcast. 5 * 6 * @return string 7 */ 8public function broadcastType() 9{10 return 'broadcast.message';11}
Listening For Notifications
Notifications will broadcast on a private channel formatted using a {notifiable}.{id}
convention. So, if you are sending a notification to an App\Models\User
instance with an ID of 1
, the notification will be broadcast on the App.Models.User.1
private channel. When using Laravel Echo, you may easily listen for notifications on a channel using the notification
method:
1Echo.private('App.Models.User.' + userId)2 .notification((notification) => {3 console.log(notification.type);4 });
Customizing The Notification Channel
If you would like to customize which channel that an entity's broadcast notifications are broadcast on, you may define a receivesBroadcastNotificationsOn
method on the notifiable entity:
1<?php 2 3namespace App\Models; 4 5use Illuminate\Broadcasting\PrivateChannel; 6use Illuminate\Foundation\Auth\User as Authenticatable; 7use Illuminate\Notifications\Notifiable; 8 9class User extends Authenticatable10{11 use Notifiable;12 13 /**14 * The channels the user receives notification broadcasts on.15 *16 * @return string17 */18 public function receivesBroadcastNotificationsOn()19 {20 return 'users.'.$this->id;21 }22}
SMS Notifications
Prerequisites
Sending SMS notifications in Laravel is powered by Vonage (formerly known as Nexmo). Before you can send notifications via Vonage, you need to install the laravel/vonage-notification-channel
and guzzlehttp/guzzle
packages:
1composer require laravel/vonage-notification-channel guzzlehttp/guzzle
The package includes a configuration file. However, you are not required to export this configuration file to your own application. You can simply use the VONAGE_KEY
and VONAGE_SECRET
environment variables to define your Vonage public and secret keys.
After defining your keys, you should set a VONAGE_SMS_FROM
environment variable that defines the phone number that your SMS messages should be sent from by default. You may generate this phone number within the Vonage control panel:
1VONAGE_SMS_FROM=15556666666
Formatting SMS Notifications
If a notification supports being sent as an SMS, you should define a toVonage
method on the notification class. This method will receive a $notifiable
entity and should return an Illuminate\Notifications\Messages\VonageMessage
instance:
1/** 2 * Get the Vonage / SMS representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\VonageMessage 6 */ 7public function toVonage($notifiable) 8{ 9 return (new VonageMessage)10 ->content('Your SMS message content');11}
Unicode Content
If your SMS message will contain unicode characters, you should call the unicode
method when constructing the VonageMessage
instance:
1/** 2 * Get the Vonage / SMS representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\VonageMessage 6 */ 7public function toVonage($notifiable) 8{ 9 return (new VonageMessage)10 ->content('Your unicode message')11 ->unicode();12}
Customizing The "From" Number
If you would like to send some notifications from a phone number that is different from the phone number specified by your VONAGE_SMS_FROM
environment variable, you may call the from
method on a VonageMessage
instance:
1/** 2 * Get the Vonage / SMS representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\VonageMessage 6 */ 7public function toVonage($notifiable) 8{ 9 return (new VonageMessage)10 ->content('Your SMS message content')11 ->from('15554443333');12}
Adding a Client Reference
If you would like to keep track of costs per user, team, or client, you may add a "client reference" to the notification. Vonage will allow you to generate reports using this client reference so that you can better understand a particular customer's SMS usage. The client reference can be any string up to 40 characters:
1/** 2 * Get the Vonage / SMS representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\VonageMessage 6 */ 7public function toVonage($notifiable) 8{ 9 return (new VonageMessage)10 ->clientReference((string) $notifiable->id)11 ->content('Your SMS message content');12}
Routing SMS Notifications
To route Vonage notifications to the proper phone number, define a routeNotificationForVonage
method on your notifiable entity:
1<?php 2 3namespace App\Models; 4 5use Illuminate\Foundation\Auth\User as Authenticatable; 6use Illuminate\Notifications\Notifiable; 7 8class User extends Authenticatable 9{10 use Notifiable;11 12 /**13 * Route notifications for the Vonage channel.14 *15 * @param \Illuminate\Notifications\Notification $notification16 * @return string17 */18 public function routeNotificationForVonage($notification)19 {20 return $this->phone_number;21 }22}
Slack Notifications
Prerequisites
Before you can send notifications via Slack, you must install the Slack notification channel via Composer:
1composer require laravel/slack-notification-channel
You will also need to create a Slack App for your team. After creating the App, you should configure an "Incoming Webhook" for the workspace. Slack will then provide you with a webhook URL that you may use when routing Slack notifications.
Formatting Slack Notifications
If a notification supports being sent as a Slack message, you should define a toSlack
method on the notification class. This method will receive a $notifiable
entity and should return an Illuminate\Notifications\Messages\SlackMessage
instance. Slack messages may contain text content as well as an "attachment" that formats additional text or an array of fields. Let's take a look at a basic toSlack
example:
1/** 2 * Get the Slack representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\SlackMessage 6 */ 7public function toSlack($notifiable) 8{ 9 return (new SlackMessage)10 ->content('One of your invoices has been paid!');11}
Slack Attachments
You may also add "attachments" to Slack messages. Attachments provide richer formatting options than simple text messages. In this example, we will send an error notification about an exception that occurred in an application, including a link to view more details about the exception:
1/** 2 * Get the Slack representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return \Illuminate\Notifications\Messages\SlackMessage 6 */ 7public function toSlack($notifiable) 8{ 9 $url = url('/exceptions/'.$this->exception->id);10 11 return (new SlackMessage)12 ->error()13 ->content('Whoops! Something went wrong.')14 ->attachment(function ($attachment) use ($url) {15 $attachment->title('Exception: File Not Found', $url)16 ->content('File [background.jpg] was not found.');17 });18}
Attachments also allow you to specify an array of data that should be presented to the user. The given data will be presented in a table-style format for easy reading:
1/** 2 * Get the Slack representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return SlackMessage 6 */ 7public function toSlack($notifiable) 8{ 9 $url = url('/invoices/'.$this->invoice->id);10 11 return (new SlackMessage)12 ->success()13 ->content('One of your invoices has been paid!')14 ->attachment(function ($attachment) use ($url) {15 $attachment->title('Invoice 1322', $url)16 ->fields([17 'Title' => 'Server Expenses',18 'Amount' => '$1,234',19 'Via' => 'American Express',20 'Was Overdue' => ':-1:',21 ]);22 });23}
Markdown Attachment Content
If some of your attachment fields contain Markdown, you may use the markdown
method to instruct Slack to parse and display the given attachment fields as Markdown formatted text. The values accepted by this method are: pretext
, text
, and / or fields
. For more information about Slack attachment formatting, check out the Slack API documentation:
1/** 2 * Get the Slack representation of the notification. 3 * 4 * @param mixed $notifiable 5 * @return SlackMessage 6 */ 7public function toSlack($notifiable) 8{ 9 $url = url('/exceptions/'.$this->exception->id);10 11 return (new SlackMessage)12 ->error()13 ->content('Whoops! Something went wrong.')14 ->attachment(function ($attachment) use ($url) {15 $attachment->title('Exception: File Not Found', $url)16 ->content('File [background.jpg] was *not found*.')17 ->markdown(['text']);18 });19}
Routing Slack Notifications
To route Slack notifications to the proper Slack team and channel, define a routeNotificationForSlack
method on your notifiable entity. This should return the webhook URL to which the notification should be delivered. Webhook URLs may be generated by adding an "Incoming Webhook" service to your Slack team:
1<?php 2 3namespace App\Models; 4 5use Illuminate\Foundation\Auth\User as Authenticatable; 6use Illuminate\Notifications\Notifiable; 7 8class User extends Authenticatable 9{10 use Notifiable;11 12 /**13 * Route notifications for the Slack channel.14 *15 * @param \Illuminate\Notifications\Notification $notification16 * @return string17 */18 public function routeNotificationForSlack($notification)19 {20 return 'https://hooks.slack.com/services/...';21 }22}
Localizing Notifications
Laravel allows you to send notifications in a locale other than the HTTP request's current locale, and will even remember this locale if the notification is queued.
To accomplish this, the Illuminate\Notifications\Notification
class offers a locale
method to set the desired language. The application will change into this locale when the notification is being evaluated and then revert back to the previous locale when evaluation is complete:
1$user->notify((new InvoicePaid($invoice))->locale('es'));
Localization of multiple notifiable entries may also be achieved via the Notification
facade:
1Notification::locale('es')->send(2 $users, new InvoicePaid($invoice)3);
User Preferred Locales
Sometimes, applications store each user's preferred locale. By implementing the HasLocalePreference
contract on your notifiable model, you may instruct Laravel to use this stored locale when sending a notification:
1use Illuminate\Contracts\Translation\HasLocalePreference; 2 3class User extends Model implements HasLocalePreference 4{ 5 /** 6 * Get the user's preferred locale. 7 * 8 * @return string 9 */10 public function preferredLocale()11 {12 return $this->locale;13 }14}
Once you have implemented the interface, Laravel will automatically use the preferred locale when sending notifications and mailables to the model. Therefore, there is no need to call the locale
method when using this interface:
1$user->notify(new InvoicePaid($invoice));
Notification Events
Notification Sending Event
When a notification is sending, the Illuminate\Notifications\Events\NotificationSending
event is dispatched by the notification system. This contains the "notifiable" entity and the notification instance itself. You may register listeners for this event in your application's EventServiceProvider
:
1use App\Listeners\CheckNotificationStatus; 2use Illuminate\Notifications\Events\NotificationSending; 3 4/** 5 * The event listener mappings for the application. 6 * 7 * @var array 8 */ 9protected $listen = [10 NotificationSending::class => [11 CheckNotificationStatus::class,12 ],13];
The notification will not be sent if an event listener for the NotificationSending
event returns false
from its handle
method:
1use Illuminate\Notifications\Events\NotificationSending; 2 3/** 4 * Handle the event. 5 * 6 * @param \Illuminate\Notifications\Events\NotificationSending $event 7 * @return void 8 */ 9public function handle(NotificationSending $event)10{11 return false;12}
Within an event listener, you may access the notifiable
, notification
, and channel
properties on the event to learn more about the notification recipient or the notification itself:
1/** 2 * Handle the event. 3 * 4 * @param \Illuminate\Notifications\Events\NotificationSending $event 5 * @return void 6 */ 7public function handle(NotificationSending $event) 8{ 9 // $event->channel10 // $event->notifiable11 // $event->notification12}
Notification Sent Event
When a notification is sent, the Illuminate\Notifications\Events\NotificationSent
event is dispatched by the notification system. This contains the "notifiable" entity and the notification instance itself. You may register listeners for this event in your EventServiceProvider
:
1use App\Listeners\LogNotification; 2use Illuminate\Notifications\Events\NotificationSent; 3 4/** 5 * The event listener mappings for the application. 6 * 7 * @var array 8 */ 9protected $listen = [10 NotificationSent::class => [11 LogNotification::class,12 ],13];
After registering listeners in your EventServiceProvider
, use the event:generate
Artisan command to quickly generate listener classes.
Within an event listener, you may access the notifiable
, notification
, channel
, and response
properties on the event to learn more about the notification recipient or the notification itself:
1/** 2 * Handle the event. 3 * 4 * @param \Illuminate\Notifications\Events\NotificationSent $event 5 * @return void 6 */ 7public function handle(NotificationSent $event) 8{ 9 // $event->channel10 // $event->notifiable11 // $event->notification12 // $event->response13}
Custom Channels
Laravel ships with a handful of notification channels, but you may want to write your own drivers to deliver notifications via other channels. Laravel makes it simple. To get started, define a class that contains a send
method. The method should receive two arguments: a $notifiable
and a $notification
.
Within the send
method, you may call methods on the notification to retrieve a message object understood by your channel and then send the notification to the $notifiable
instance however you wish:
1<?php 2 3namespace App\Notifications; 4 5use Illuminate\Notifications\Notification; 6 7class VoiceChannel 8{ 9 /**10 * Send the given notification.11 *12 * @param mixed $notifiable13 * @param \Illuminate\Notifications\Notification $notification14 * @return void15 */16 public function send($notifiable, Notification $notification)17 {18 $message = $notification->toVoice($notifiable);19 20 // Send notification to the $notifiable instance...21 }22}
Once your notification channel class has been defined, you may return the class name from the via
method of any of your notifications. In this example, the toVoice
method of your notification can return whatever object you choose to represent voice messages. For example, you might define your own VoiceMessage
class to represent these messages:
1<?php 2 3namespace App\Notifications; 4 5use App\Notifications\Messages\VoiceMessage; 6use App\Notifications\VoiceChannel; 7use Illuminate\Bus\Queueable; 8use Illuminate\Contracts\Queue\ShouldQueue; 9use Illuminate\Notifications\Notification;10 11class InvoicePaid extends Notification12{13 use Queueable;14 15 /**16 * Get the notification channels.17 *18 * @param mixed $notifiable19 * @return array|string20 */21 public function via($notifiable)22 {23 return [VoiceChannel::class];24 }25 26 /**27 * Get the voice representation of the notification.28 *29 * @param mixed $notifiable30 * @return VoiceMessage31 */32 public function toVoice($notifiable)33 {34 // ...35 }36}